CORS error prevention in AWS Serverless configuration
When I was developing my personal web application , I got a CORS error when I wanted to hit the deployed Backend API during Frontend development, and it was a bit troublesome, so I wrote down the solution.
What is CORS?
As you can see, CORS stands for Cross-Origin Resource Sharing, which means that resources are shared between different domains. To be precise, it also includes port numbers, so it means sharing between different programs as well. To put it simply, a web app called http://1.com:80 from a web app called http://2.com:80 to a server called http://2.com:80 from a web app called to a resource on a server called . Basically, sharing resources between different origins in this way is restricted, and if you don’t think about it and include it in your code, the browser will return an error.
In my case, it happened when I made an HTTP request from http://localhost:3000
, which is being developed locally, to https://app.wayama.io/api/v1/xxxxxx
, which is running in production. Also, since this system is implemented as a Serverless application in AWS, it was necessary to configure CORS according to AWS rules.
Server configuration
The architectural configuration of the AWS Frontend and Backend is as follows: the use case is to use the API already deployed on the server for Frontend development. The domain of the Front is https://app.wayama.io/api/v1/xxxxxx
and the domain of the API of the Backend is https://app.wayama.io/api/v1/xxxxxx
.
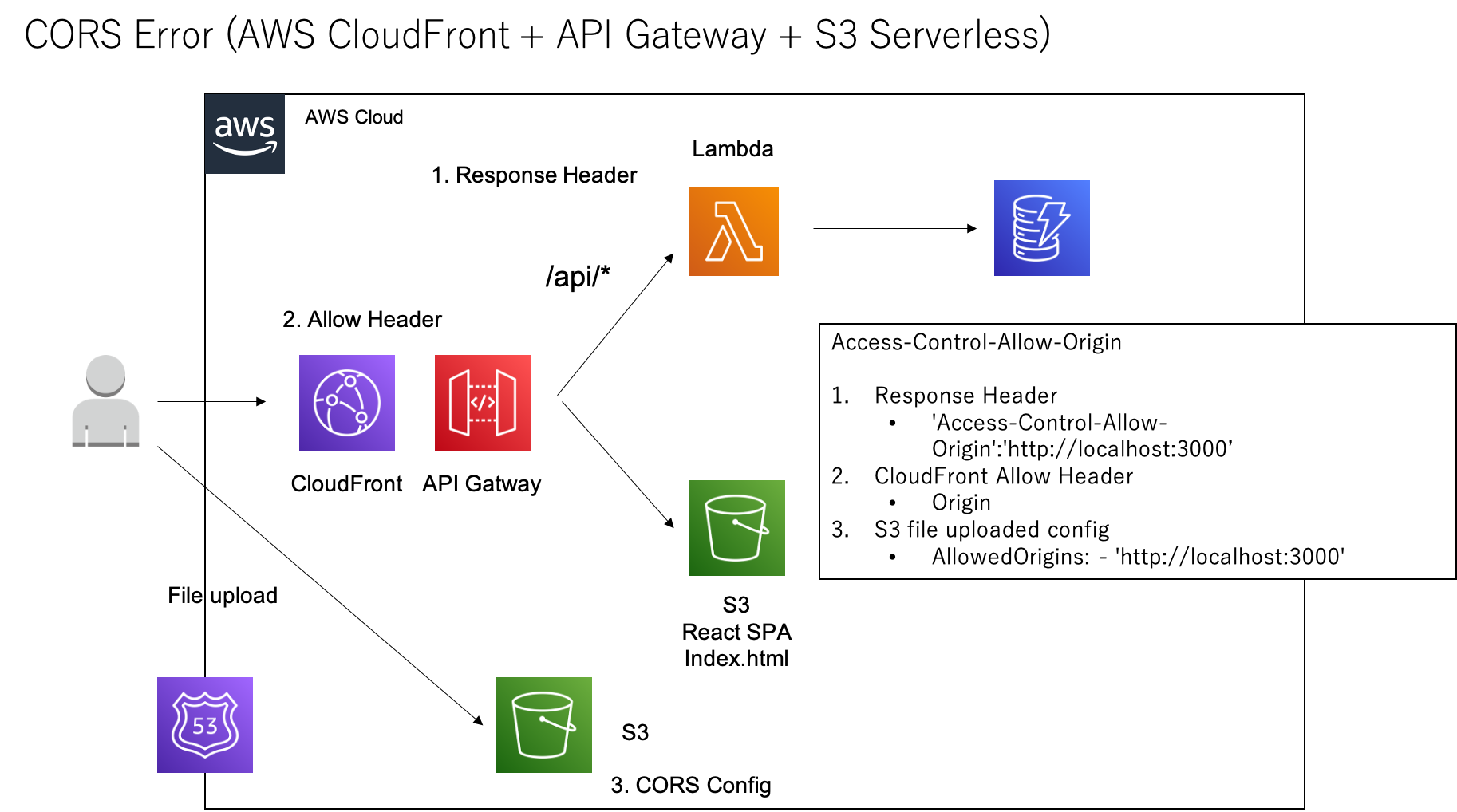
Frontend.
Develop Frontend.
- Domain :
http://localhost:3000
- javascript (react)
- AWS CloudFront + APIGateway + S3 (Serverless)
Backend
I want to use the API of Backend when debugging Frontend development.
- Domain :
https://app.wayama.io/api/v1/xxxxxx
- python
- AWS CloudFront + APIGateway + Lambda
What you need to set up.
- set the response header of the API
- cloudfront allow header setting Set up the Cors of the S3 to upload to.
1. response header setting
You need to set Access-Control-Allow-Origin in the API response header.
{
'Content-Type': 'application/json',
'Access-Control-Allow-Origin': 'http://localhost:3000',
}
2. Configure cloudfront’s Whitelist Headers
In order for Cloudfront to forward the header named Origin to the later APIGateway, we need to register the whitelist.
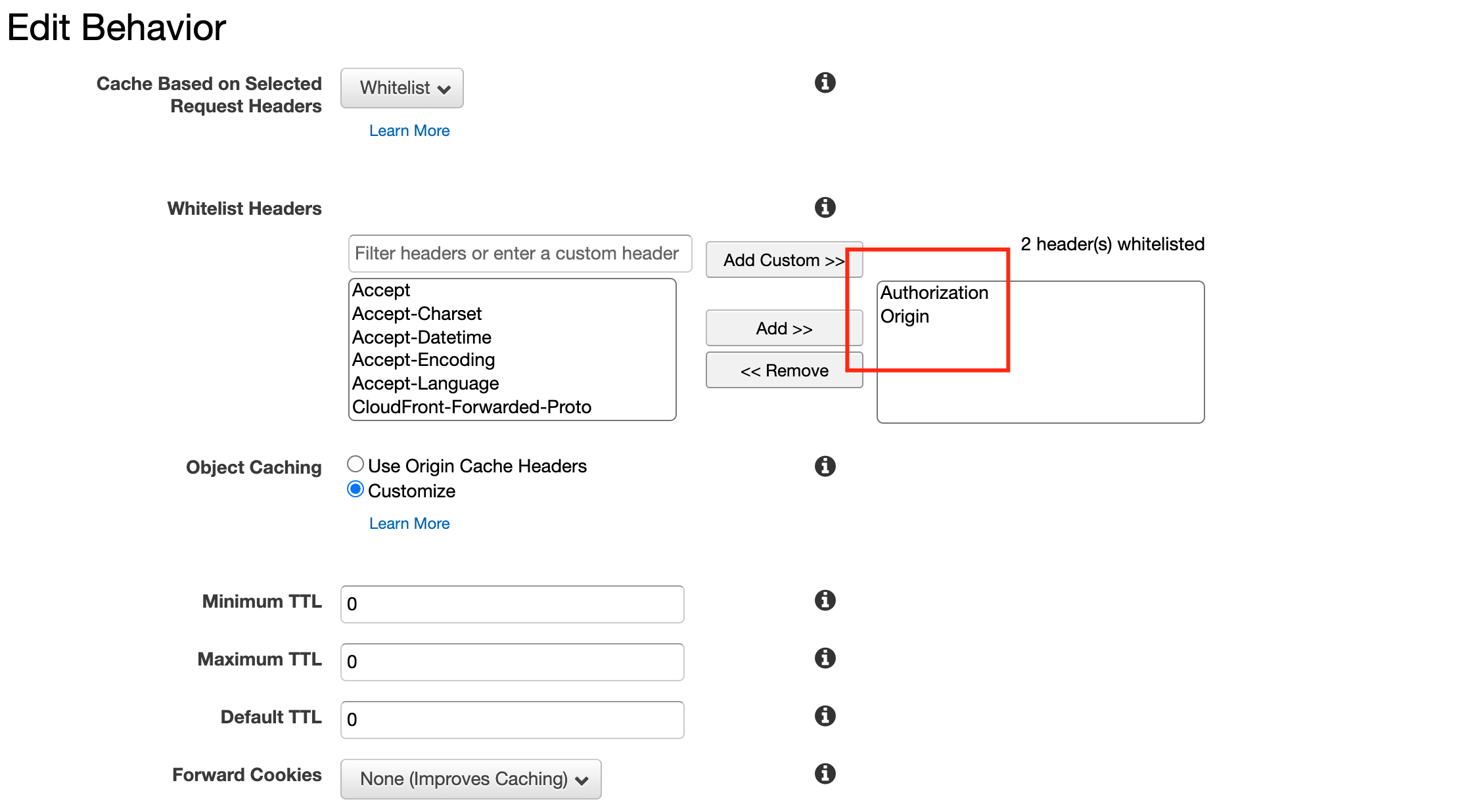
3. CloudFormation template of S3 for Upload destination
You need to set http://localhost:3000
as AllowOrigin in S3’s CORS settings.
CorsConfiguration:
CorsRules:
- AllowedHeaders:
- '*'.
AllowedMethods:
- GET
- POST
- PUT
AllowedOrigins:
- 'http://localhost:3000'
- 'https://app.wayama.io'
ExposedHeaders:
- Date
MaxAge: '3600'.
Conclusion
It is too inefficient to check the behavior of Front without deploying it to S3 each time. We can now develop more efficiently.